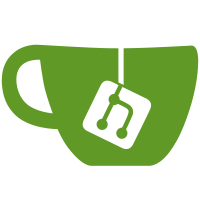
While investigating something else in Shared, I noticed that CodePage had poor test coverage and a high complexity rating. This change addresses both; Scrutinizer would love it, although its interface on GitHub seems broken at the moment (all PRs show "Waiting for External Code Coverage").
71 lines
1.8 KiB
PHP
71 lines
1.8 KiB
PHP
<?php
|
|
|
|
namespace PhpOffice\PhpSpreadsheetTests\Shared;
|
|
|
|
use PhpOffice\PhpSpreadsheet\Exception;
|
|
use PhpOffice\PhpSpreadsheet\Shared\CodePage;
|
|
use PHPUnit\Framework\TestCase;
|
|
|
|
class CodePageTest extends TestCase
|
|
{
|
|
/**
|
|
* @dataProvider providerCodePage
|
|
*
|
|
* @param mixed $expectedResult
|
|
*/
|
|
public function testCodePageNumberToName($expectedResult, ...$args): void
|
|
{
|
|
$result = CodePage::numberToName(...$args);
|
|
self::assertEquals($expectedResult, $result);
|
|
}
|
|
|
|
public function providerCodePage()
|
|
{
|
|
return require 'tests/data/Shared/CodePage.php';
|
|
}
|
|
|
|
public function testCoverage(): void
|
|
{
|
|
$covered = [];
|
|
$expected = CodePage::getEncodings();
|
|
foreach ($expected as $key => $val) {
|
|
$covered[$key] = 0;
|
|
}
|
|
$tests = $this->providerCodePage();
|
|
foreach ($tests as $test) {
|
|
$covered[$test[1]] = 1;
|
|
}
|
|
foreach ($covered as $key => $val) {
|
|
self::assertEquals(1, $val, "Codepage $key not tested");
|
|
}
|
|
}
|
|
|
|
public function testNumberToNameWithInvalidCodePage(): void
|
|
{
|
|
$invalidCodePage = 12345;
|
|
|
|
try {
|
|
CodePage::numberToName($invalidCodePage);
|
|
} catch (Exception $e) {
|
|
self::assertEquals($e->getMessage(), 'Unknown codepage: 12345');
|
|
|
|
return;
|
|
}
|
|
self::fail('An expected exception has not been raised.');
|
|
}
|
|
|
|
public function testNumberToNameWithUnsupportedCodePage(): void
|
|
{
|
|
$unsupportedCodePage = 720;
|
|
|
|
try {
|
|
CodePage::numberToName($unsupportedCodePage);
|
|
} catch (Exception $e) {
|
|
self::assertEquals($e->getMessage(), 'Code page 720 not supported.');
|
|
|
|
return;
|
|
}
|
|
self::fail('An expected exception has not been raised.');
|
|
}
|
|
}
|